C#.Net组件开发(高级篇) - 使用自定义TypeConverter生成设计时代码

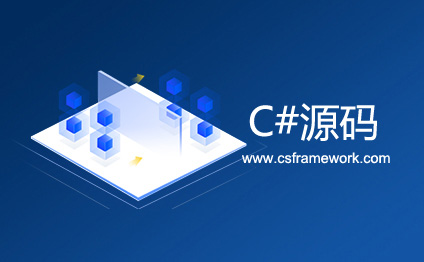
拖放一个TestComponent组件到窗体Form1上,按F4弹出属性窗体在MyPoint,Persion内输入字符串,设置如下:
在Form1.Designer.cs文件内生成设计时代码。
测试用的Component组件:
C# Code:
/// <summary>
/// 测试用的Component组件
/// </summary>
public partial class TestComponent : Component
{
//使用.Net自带的InstanceDescriptor生成设计时代码
private Guid _MyID;
//使用自定义TypeConverter生成设计时代码
private MyPoint _MyPoint;
//使用自定义TypeConverter生成设计时代码
private Person _Person;
[Browsable(true)]
public Guid MyID
{
get { return _MyID; }
set { _MyID = value; }
}
[Browsable(true)]
public MyPoint MyPoint
{
get { return _MyPoint; }
set { _MyPoint = value; }
}
[Browsable(true)]
public Person Person
{
get
{
return _Person;
}
set
{
this._Person = value;
}
}
}
//来源:C/S框架网(www.csframework.com) QQ:1980854898
/// <summary>
/// 测试用的Component组件
/// </summary>
public partial class TestComponent : Component
{
//使用.Net自带的InstanceDescriptor生成设计时代码
private Guid _MyID;
//使用自定义TypeConverter生成设计时代码
private MyPoint _MyPoint;
//使用自定义TypeConverter生成设计时代码
private Person _Person;
[Browsable(true)]
public Guid MyID
{
get { return _MyID; }
set { _MyID = value; }
}
[Browsable(true)]
public MyPoint MyPoint
{
get { return _MyPoint; }
set { _MyPoint = value; }
}
[Browsable(true)]
public Person Person
{
get
{
return _Person;
}
set
{
this._Person = value;
}
}
}
//来源:C/S框架网(www.csframework.com) QQ:1980854898
测试用的对象,用于生成设计时代码:
C# Code:
/// <summary>
/// 生成设计时代码的对象
/// </summary>
[TypeConverter(typeof(PersonConverter))] //自定义类型转换器
//[TypeConverter(typeof(PersonConverterExpandable))]//试验第二种类型转换器
public class Person
{
private string _FirstName = "";
private string _LastName = "";
private int _Age = 0;
public Person(string firstName, string lastName, int age)
{
_FirstName = firstName;
_LastName = lastName;
_Age = age;
}
public int Age
{
get { return _Age; }
set { _Age = value; }
}
public string FirstName
{
get { return _FirstName; }
set { _FirstName = value; }
}
public string LastName
{
get { return _LastName; }
set { _LastName = value; }
}
}
//来源:C/S框架网(www.csframework.com) QQ:1980854898
/// <summary>
/// 生成设计时代码的对象
/// </summary>
[TypeConverter(typeof(PersonConverter))] //自定义类型转换器
//[TypeConverter(typeof(PersonConverterExpandable))]//试验第二种类型转换器
public class Person
{
private string _FirstName = "";
private string _LastName = "";
private int _Age = 0;
public Person(string firstName, string lastName, int age)
{
_FirstName = firstName;
_LastName = lastName;
_Age = age;
}
public int Age
{
get { return _Age; }
set { _Age = value; }
}
public string FirstName
{
get { return _FirstName; }
set { _FirstName = value; }
}
public string LastName
{
get { return _LastName; }
set { _LastName = value; }
}
}
//来源:C/S框架网(www.csframework.com) QQ:1980854898
Person对象的类型转换器实现:
C# Code:
/// <summary>
/// Person对象的类型转换器实现
/// </summary>
public class PersonConverter : TypeConverter
{
public PersonConverter() { }
public override bool CanConvertFrom(ITypeDescriptorContext context,
Type sourceType)
{
if (sourceType == typeof(string)) return true;//字符串,如:"Jonny,Sun,33"
return base.CanConvertFrom(context, sourceType);
}
public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType)
{
if (destinationType == typeof(string)) return true;
if (destinationType == typeof(InstanceDescriptor)) return true;
return base.CanConvertTo(context, destinationType);
}
public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value)
{
string s = value as string;
if (s == null) return base.ConvertFrom(context, culture, value);
//字符串,如:"Jonny,Sun,33"
string[] ps = s.Split(new char[] { char.Parse(",") });
if (ps.Length != 3)
throw new ArgumentException("Failed to parse Text(" s
") expected text in the format \"FirstName,LastName,Age.\"");
//解析字符串并实例化对象
return new Person(ps[0], ps[1], int.Parse(ps[2]));
}
public override object ConvertTo(ITypeDescriptorContext context,
CultureInfo culture,
object value,
Type destinationType)
{
//将对象转换为字符串,如:"Jonny,Sun,33"
if ((destinationType == typeof(string)) &&& (value is Person))
return ((Person)value).FirstName "," ((Person)value).LastName "," ((Person)value).Age.ToString();
//生成设计时的构造器代码
// this.testComponent1.Person = new CSFramework.MyTypeConverter.Person("Jonny", "Sun", 33);
if (destinationType == typeof(InstanceDescriptor) & value is Person)
{
Person p = (Person)value;
ConstructorInfo ctor = typeof(Person).GetConstructor(
new Type[] { typeof(string), typeof(string), typeof(int) });
return new InstanceDescriptor(ctor, new object[] { p.FirstName, p.LastName, p.Age });
}
return base.ConvertTo(context, culture, value, destinationType);
}
public override object CreateInstance(ITypeDescriptorContext context,
IDictionary propertyValues)
{
string s1 = (string)propertyValues["FirstName"];
string s2 = (string)propertyValues["LastName"];
int age = (int)propertyValues["Age"];
return new Person(s1, s2, age);//创建实例
}
public override PropertyDescriptorCollection GetProperties(
ITypeDescriptorContext context,
object value, Attribute[] attributes)
{
if (value is Person)
return TypeDescriptor.GetProperties(value, attributes);
return base.GetProperties(context, value, attributes);
}
public override bool GetCreateInstanceSupported(ITypeDescriptorContext context)
{
return true;
}
public override bool GetPropertiesSupported(ITypeDescriptorContext context)
{
return true;
}
}
//来源:C/S框架网(www.csframework.com) QQ:1980854898
/// <summary>
/// Person对象的类型转换器实现
/// </summary>
public class PersonConverter : TypeConverter
{
public PersonConverter() { }
public override bool CanConvertFrom(ITypeDescriptorContext context,
Type sourceType)
{
if (sourceType == typeof(string)) return true;//字符串,如:"Jonny,Sun,33"
return base.CanConvertFrom(context, sourceType);
}
public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType)
{
if (destinationType == typeof(string)) return true;
if (destinationType == typeof(InstanceDescriptor)) return true;
return base.CanConvertTo(context, destinationType);
}
public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value)
{
string s = value as string;
if (s == null) return base.ConvertFrom(context, culture, value);
//字符串,如:"Jonny,Sun,33"
string[] ps = s.Split(new char[] { char.Parse(",") });
if (ps.Length != 3)
throw new ArgumentException("Failed to parse Text(" s
") expected text in the format \"FirstName,LastName,Age.\"");
//解析字符串并实例化对象
return new Person(ps[0], ps[1], int.Parse(ps[2]));
}
public override object ConvertTo(ITypeDescriptorContext context,
CultureInfo culture,
object value,
Type destinationType)
{
//将对象转换为字符串,如:"Jonny,Sun,33"
if ((destinationType == typeof(string)) &&& (value is Person))
return ((Person)value).FirstName "," ((Person)value).LastName "," ((Person)value).Age.ToString();
//生成设计时的构造器代码
// this.testComponent1.Person = new CSFramework.MyTypeConverter.Person("Jonny", "Sun", 33);
if (destinationType == typeof(InstanceDescriptor) & value is Person)
{
Person p = (Person)value;
ConstructorInfo ctor = typeof(Person).GetConstructor(
new Type[] { typeof(string), typeof(string), typeof(int) });
return new InstanceDescriptor(ctor, new object[] { p.FirstName, p.LastName, p.Age });
}
return base.ConvertTo(context, culture, value, destinationType);
}
public override object CreateInstance(ITypeDescriptorContext context,
IDictionary propertyValues)
{
string s1 = (string)propertyValues["FirstName"];
string s2 = (string)propertyValues["LastName"];
int age = (int)propertyValues["Age"];
return new Person(s1, s2, age);//创建实例
}
public override PropertyDescriptorCollection GetProperties(
ITypeDescriptorContext context,
object value, Attribute[] attributes)
{
if (value is Person)
return TypeDescriptor.GetProperties(value, attributes);
return base.GetProperties(context, value, attributes);
}
public override bool GetCreateInstanceSupported(ITypeDescriptorContext context)
{
return true;
}
public override bool GetPropertiesSupported(ITypeDescriptorContext context)
{
return true;
}
}
//来源:C/S框架网(www.csframework.com) QQ:1980854898

扫一扫加作者微信
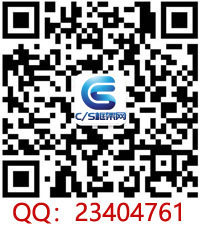
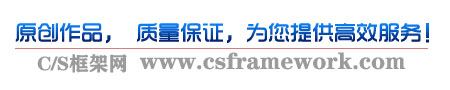
版权声明:本文为开发框架文库发布内容,转载请附上原文出处连接
NewDoc C/S框架网