ASP.NET MVC中几种常用的ActionResult详解

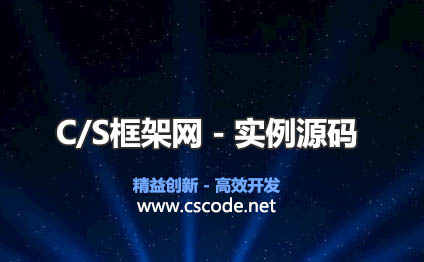
一、定义
MVC中ActionResult是Action的返回结果。ActionResult 有多个派生类,每个子类功能均不同,并不是所有的子类都需要返回视图View,有些直接返回流,有些返回字符串等。ActionResult是一个抽象类,它定义了唯一的ExecuteResult方法,参数为一个ControllerContext,下面为您介绍MVC中的ActionResult 的用法
二、什么是ActionResult
ActionResult是控制器方法执行后返回的结果类型,控制器方法可以返回一个直接或间接从ActionResult抽象类继承的类型,如果返回的 是非ActionResult类型,控制器将会将结果转换为一个ContentResult类型。默认的ControllerActionInvoker 调用ActionResult.ExecuteResult方法生成应答结果。
三、常见的ActionResult
1、ViewResult
表示一个视图结果,它根据视图模板产生应答内容。对应得Controller方法为View。
2、PartialViewResult
表示一个部分视图结果,与ViewResult本质上一致,只是部分视图不支持母版,对应于ASP.NET,ViewResult相当于一个Page,而PartialViewResult 则相当于一个UserControl。它对应得Controller方法的PartialView.
3、RedirectResult
表示一个连接跳转,相当于ASP.NET中的Response.Redirect方法,对应得Controller方法为Redirect。
4、RedirectToRouteResult
同样表示一个跳转,MVC会根据我们指定的路由名称或路由信息(RouteValueDictionary)来生成Url地址,然后调用Response.Redirect跳转。对应的Controller方法为RedirectToAction和RedirectToRoute.
5、ContentResult
返回简单的纯文本内容,可通过ContentType属性指定应答文档类型,通过ContentEncoding属性指定应答文档的字符编码。可通过Controller类中的Content方法便捷地返回ContentResult对象。如果控制器方法返回非ActionResult对象,MVc将简单地以返回对象的toString()内容为基础产生一个ContentResult对象。
6、EmptyResult
返回一个空的结果,如果控制器方法返回一个null ,MVC将其转换成EmptyResult对象。
7、JavaScriptResult
本质上是一个文本内容,只是将Response.ContentType设置为application/x-javascript,此结果应该和MicrosoftMvcAjax.js脚本配合使用,客户端接收到Ajax应答后,将判断Response.ContentType的值,如果是application/x-javascript,则直接eval 执行返回的应答内容,此结果类型对应得Controller方法为JavaScript.
8、JsonResult
表示一个Json结果。MVC将Response.ContentType 设置为application/json,并通过JavaScriptSerializer类指定对象序列化为Json表示方式。需要注意,默认情况下,Mvc不允许GET请求返回Json结果,要解除此限制,在生成JsonResult对象时,将其JsonRequestBehavior属性设置为JsonRequestBehavior.AllowGet,此结果对应Controller方法的Json.
9、FileResult(FilePathResult、FileContentResult、FileStreamResult)
这三个类继承于FileResult,表示一个文件内容,三者区别在于,FilePath 通过路径传送文件到客户端,FileContent 通过二进制数据的方式,而FileStream 是通过Stream(流)的方式来传送。Controller为这三个文件结果类型提供了一个名为File的重载方法。
FilePathResult: 直接将一个文件发送给客户端
FileContentResult: 返回byte字节给客户端(比如图片)
FileStreamResult: 返回流
10、HttpUnauthorizedResult
表示一个未经授权访问的错误,MVC会向客户端发送一个401的应答状态。如果在web.config 中开启了表单验证(authenication mode=”Forms”),则401状态会将Url 转向指定的loginUrl 链接。
11、HttpStatusCodeResult
返回一个服务器的错误信息
12、HttpNoFoundResult
返回一个找不到Action错误信息
四、ActionResult子类之间的关系表
.png)
五、ActionResult(12种)的简单应用
源码:
C# Code:
using StudyMVC4.Models;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using System.Web.Mvc;
namespace StudyMVC4.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
/// <summary>
/// ContentResult用法(返回文本)
/// http://localhost:30735/home/ContentResultDemo
/// </summary>
/// <returns>返回文本</returns>
public ActionResult ContentResultDemo()
{
string str = "ContentResultDemo!";
return Content(str);
}
/// <summary>
/// EmptyResult的用法(返回空对象)
/// http://localhost:30735/home/EmptyResultDemo
/// </summary>
/// <returns>返回一个空对象</returns>
public ActionResult EmptyResultDemo()
{
return new EmptyResult();
}
/// <summary>
/// FileContentResult的用法(返回图片)
/// http://localhost:30735/home/FileContentResultDemo
/// </summary>
/// <returns>显示一个文件内容</returns>
public ActionResult FileContentResultDemo()
{
FileStream fs = new FileStream(Server.MapPath(@"/Images/001.jpg"), FileMode.Open, FileAccess.Read);
byte[] buffer = new byte[Convert.ToInt32(fs.Length)];
fs.Read(buffer, 0, Convert.ToInt32(fs.Length));
string contentType = "image/jpeg";
return File(buffer, contentType);
}
/// <summary>
/// FilePathResult的用法(返回图片)
/// http://localhost:30735/home/FilePathResultDemo/002
/// </summary>
/// <param name="id">图片id</param>
/// <returns>直接将返回一个文件对象</returns>
public FilePathResult FilePathResultDemo(string id)
{
string path = Server.MapPath(@"/Images/" + id + ".jpg");
//定义内容类型(图片)
string contentType = "image/jpeg";
//FilePathResult直接返回file对象
return File(path, contentType);
}
/// <summary>
/// FileStreamResult的用法(返回图片)
/// http://localhost:30735/home/FileStreamResultDemo
/// </summary>
/// <returns>返回文件流(图片)</returns>
public ActionResult FileStreamResultDemo()
{
FileStream fs = new FileStream(Server.MapPath(@"/Images/001.jpg"), FileMode.Open, FileAccess.Read);
string contentType = "image/jpeg";
return File(fs, contentType);
}
/// <summary>
/// HttpUnauthorizedResult 的用法(抛出401错误)
/// http://localhost:30735/home/HttpUnauthorizedResult
/// </summary>
/// <returns></returns>
public ActionResult HttpUnauthorizedResultDemo()
{
return new HttpUnauthorizedResult();
}
/// <summary>
/// HttpStatusCodeResult的方法(返回错误状态信息)
/// http://localhost:30735/home/HttpStatusCodeResult
/// </summary>
/// <returns></returns>
public ActionResult HttpStatusCodeResultDemo()
{
return new HttpStatusCodeResult(500, "System Error");
}
/// <summary>
/// HttpNotFoundResult的使用方法
/// http://localhost:30735/home/HttpNotFoundResultDemo
/// </summary>
/// <returns></returns>
public ActionResult HttpNotFoundResultDemo()
{
return new HttpNotFoundResult("not found action");
}
/// <summary>
/// JavaScriptResult 的用法(返回脚本文件)
/// http://localhost:30735/home/JavaScriptResultDemo
/// </summary>
/// <returns>返回脚本内容</returns>
public ActionResult JavaScriptResultDemo()
{
return JavaScript(@"<script>alert('Test JavaScriptResultDemo!')</script>");
}
/// <summary>
/// JsonResult的用法(返回一个json对象)
/// http://localhost:30735/home/JsonResultDemo
/// </summary>
/// <returns>返回一个json对象</returns>
public ActionResult JsonResultDemo()
{
var tempObj = new { Controller = "HomeController", Action = "JsonResultDemo" };
return Json(tempObj);
}
/// <summary>
/// RedirectResult的用法(跳转url地址)
/// http://localhost:30735/home/RedirectResultDemo
/// </summary>
/// <returns></returns>
public ActionResult RedirectResultDemo()
{
return Redirect(@"http://wwww.baidu.com");
}
/// <summary>
/// RedirectToRouteResult的用法(跳转的action名称)
/// http://localhost:30735/home/RedirectToRouteResultDemo
/// </summary>
/// <returns></returns>
public ActionResult RedirectToRouteResultDemo()
{
return RedirectToAction(@"FileStreamResultDemo");
}
/// <summary>
/// PartialViewResult的用法(返回部分视图)
/// http://localhost:30735/home/PartialViewResultDemo
/// </summary>
/// <returns></returns>
public PartialViewResult PartialViewResultDemo()
{
return PartialView();
}
/// <summary>
/// ViewResult的用法(返回视图)
/// http://localhost:30735/home/ViewResultDemo
/// </summary>
/// <returns></returns>
public ActionResult ViewResultDemo()
{
//如果没有传入View名称, 默认寻找与Action名称相同的View页面.
return View();
}
}
}
//来源:C/S框架网(www.csframework.com) QQ:23404761
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
using System.Web.Mvc;
namespace StudyMVC4.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
/// <summary>
/// ContentResult用法(返回文本)
/// http://localhost:30735/home/ContentResultDemo
/// </summary>
/// <returns>返回文本</returns>
public ActionResult ContentResultDemo()
{
string str = "ContentResultDemo!";
return Content(str);
}
/// <summary>
/// EmptyResult的用法(返回空对象)
/// http://localhost:30735/home/EmptyResultDemo
/// </summary>
/// <returns>返回一个空对象</returns>
public ActionResult EmptyResultDemo()
{
return new EmptyResult();
}
/// <summary>
/// FileContentResult的用法(返回图片)
/// http://localhost:30735/home/FileContentResultDemo
/// </summary>
/// <returns>显示一个文件内容</returns>
public ActionResult FileContentResultDemo()
{
FileStream fs = new FileStream(Server.MapPath(@"/Images/001.jpg"), FileMode.Open, FileAccess.Read);
byte[] buffer = new byte[Convert.ToInt32(fs.Length)];
fs.Read(buffer, 0, Convert.ToInt32(fs.Length));
string contentType = "image/jpeg";
return File(buffer, contentType);
}
/// <summary>
/// FilePathResult的用法(返回图片)
/// http://localhost:30735/home/FilePathResultDemo/002
/// </summary>
/// <param name="id">图片id</param>
/// <returns>直接将返回一个文件对象</returns>
public FilePathResult FilePathResultDemo(string id)
{
string path = Server.MapPath(@"/Images/" + id + ".jpg");
//定义内容类型(图片)
string contentType = "image/jpeg";
//FilePathResult直接返回file对象
return File(path, contentType);
}
/// <summary>
/// FileStreamResult的用法(返回图片)
/// http://localhost:30735/home/FileStreamResultDemo
/// </summary>
/// <returns>返回文件流(图片)</returns>
public ActionResult FileStreamResultDemo()
{
FileStream fs = new FileStream(Server.MapPath(@"/Images/001.jpg"), FileMode.Open, FileAccess.Read);
string contentType = "image/jpeg";
return File(fs, contentType);
}
/// <summary>
/// HttpUnauthorizedResult 的用法(抛出401错误)
/// http://localhost:30735/home/HttpUnauthorizedResult
/// </summary>
/// <returns></returns>
public ActionResult HttpUnauthorizedResultDemo()
{
return new HttpUnauthorizedResult();
}
/// <summary>
/// HttpStatusCodeResult的方法(返回错误状态信息)
/// http://localhost:30735/home/HttpStatusCodeResult
/// </summary>
/// <returns></returns>
public ActionResult HttpStatusCodeResultDemo()
{
return new HttpStatusCodeResult(500, "System Error");
}
/// <summary>
/// HttpNotFoundResult的使用方法
/// http://localhost:30735/home/HttpNotFoundResultDemo
/// </summary>
/// <returns></returns>
public ActionResult HttpNotFoundResultDemo()
{
return new HttpNotFoundResult("not found action");
}
/// <summary>
/// JavaScriptResult 的用法(返回脚本文件)
/// http://localhost:30735/home/JavaScriptResultDemo
/// </summary>
/// <returns>返回脚本内容</returns>
public ActionResult JavaScriptResultDemo()
{
return JavaScript(@"<script>alert('Test JavaScriptResultDemo!')</script>");
}
/// <summary>
/// JsonResult的用法(返回一个json对象)
/// http://localhost:30735/home/JsonResultDemo
/// </summary>
/// <returns>返回一个json对象</returns>
public ActionResult JsonResultDemo()
{
var tempObj = new { Controller = "HomeController", Action = "JsonResultDemo" };
return Json(tempObj);
}
/// <summary>
/// RedirectResult的用法(跳转url地址)
/// http://localhost:30735/home/RedirectResultDemo
/// </summary>
/// <returns></returns>
public ActionResult RedirectResultDemo()
{
return Redirect(@"http://wwww.baidu.com");
}
/// <summary>
/// RedirectToRouteResult的用法(跳转的action名称)
/// http://localhost:30735/home/RedirectToRouteResultDemo
/// </summary>
/// <returns></returns>
public ActionResult RedirectToRouteResultDemo()
{
return RedirectToAction(@"FileStreamResultDemo");
}
/// <summary>
/// PartialViewResult的用法(返回部分视图)
/// http://localhost:30735/home/PartialViewResultDemo
/// </summary>
/// <returns></returns>
public PartialViewResult PartialViewResultDemo()
{
return PartialView();
}
/// <summary>
/// ViewResult的用法(返回视图)
/// http://localhost:30735/home/ViewResultDemo
/// </summary>
/// <returns></returns>
public ActionResult ViewResultDemo()
{
//如果没有传入View名称, 默认寻找与Action名称相同的View页面.
return View();
}
}
}
//来源:C/S框架网(www.csframework.com) QQ:23404761
HomeController接口调用地址:
HTML Code:
http://localhost:30735/home/EmptyResultDemo
http://localhost:30735/home/ViewResultDemo
http://localhost:30735/home/ViewResultDemo
本文来源:https://www.cnblogs.com/xielong/p/5940535.html
版权声明:本文为开发框架文库发布内容,转载请附上原文出处连接
NewDoc C/S框架网