[推荐]C#图像处理(Image Processing using C#)
原文地址
示例下载
源码下载(VS2005)
简介
This is my sixth article in C#. I got impressed with a similar article, so I tried this.
概述
这篇文章的目的是构建一个图像处理功能的类而使每一个C#程序员都能够使用。因为使用C#能够非常灵活的进行图像处理。
我们知道,当基于计算来移动像素或改变像素值的代码稍微有点复杂。
应用
ImageHandler类负责图像处理的相关操作,如保存、图像关联操作等。功能包括:获取图片信息、
缩放、色彩滤镜、亮度、反向、灰度、转换、裁贴、和插入文字、其它图片或几何图形。
Paint方法使用AutoScoolPosition属性发现滚动位置,使用AutoScrollMinSize属性进行设置。
1.Color Filter(色相)
关于色相的说明:
从光学意义上讲,色相差别是由光波波长的长短产生的。即便是同一类颜色,也能分为几种色相,如黄颜色可以分为中黄、土黄、
柠檬黄等,灰颜色则可以分为红灰、蓝灰、紫灰等。光谱中有红、橙、黄、绿、蓝、紫六种基本色光,人的眼睛可以 分辨出约180种不同色相的颜色。
颜色过滤是依照颜色的光谱吸收类别:短波长、长波长、混合波长;合并或形状裁贴;单色或变色的。
详见:http://baike.baidu.com/view/122648.htm
这个比较简单,只需要增加或减少每种颜色的值即可。
使图片中的另外两种颜色值减去255即可。比如,红色相中,保持红色值不变,而对绿色和蓝色分别减去255即可。
/// 设置色相
/// </summary>
/// <param name="colorFilterType">色相类型</param>
public void SetColorFilter(ColorFilterTypes colorFilterType)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Color c;
for (int i = 0; i < bmap.Width; i++)
{
for (int j = 0; j < bmap.Height; j++)
{
c = bmap.GetPixel(i, j);
int nPixelR = 0;
int nPixelG = 0;
int nPixelB = 0;
if (colorFilterType == ColorFilterTypes.Red)
{
nPixelR = c.R;
nPixelG = c.G - 255;
nPixelB = c.B - 255;
}
else if (colorFilterType == ColorFilterTypes.Green)
{
nPixelR = c.R - 255;
nPixelG = c.G;
nPixelB = c.B - 255;
}
else if (colorFilterType == ColorFilterTypes.Blue)
{
nPixelR = c.R - 255;
nPixelG = c.G - 255;
nPixelB = c.B;
}
nPixelR = Math.Max(nPixelR, 0);
nPixelR = Math.Min(255, nPixelR);
nPixelG = Math.Max(nPixelG, 0);
nPixelG = Math.Min(255, nPixelG);
nPixelB = Math.Max(nPixelB, 0);
nPixelB = Math.Min(255, nPixelB);
bmap.SetPixel(i, j, Color.FromArgb((byte)nPixelR, (byte)nPixelG, (byte)nPixelB));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
2.Gamma(曲线)
Gamma源于CRT(显示器/电视机)的响应曲线,即其亮度与输入电压的非线性关系。
Gamma 校正补偿了不同输出设备存在的颜色显示差异,从而使图像在不同的监视器上呈现出相同的效果。
详见 :http://baike.baidu.com/view/476857.htm
/// 曲线
/// </summary>
/// <param name="red">红</param>
/// <param name="green">绿</param>
/// <param name="blue">蓝</param>
public void SetGamma(double red, double green, double blue)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Color c;
byte[] redGamma = CreateGammaArray(red);
byte[] greenGamma = CreateGammaArray(green);
byte[] blueGamma = CreateGammaArray(blue);
for (int i = 0; i < bmap.Width; i++)
{
for (int j = 0; j < bmap.Height; j++)
{
c = bmap.GetPixel(i, j);
bmap.SetPixel(i, j, Color.FromArgb(redGamma[c.R], greenGamma[c.G], blueGamma[c.B]));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
/// <summary>
/// 获取曲线数组
/// </summary>
/// <param name="color">色彩</param>
/// <returns>数组</returns>
private byte[] CreateGammaArray(double color)
{
byte[] gammaArray = new byte[256];
for (int i = 0; i < 256; ++i)
{
gammaArray[i] = (byte)Math.Min(255, (int)((255.0 * Math.Pow(i / 255.0, 1.0 / color)) + 0.5));
}
return gammaArray;
}
3. Brightness(亮度)
一种颜色的范围,表示它与一系列从很昏暗(黑)到很明亮(耀眼)的无色彩的颜色之间的相似处。
取值范围为-255到255之间。
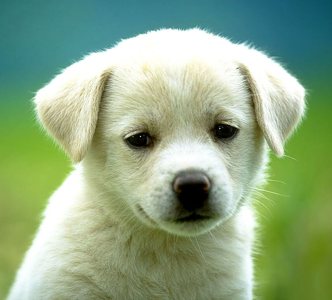
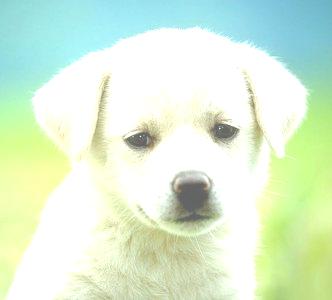
/// 设置亮度
/// </summary>
/// <param name="brightness">亮度,-255到+255之间的数值</param>
public void SetBrightness(int brightness)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
if (brightness < -255) brightness = -255;
if (brightness > 255) brightness = 255;
Color c;
for (int i = 0; i < bmap.Width; i++)
{
for (int j = 0; j < bmap.Height; j++)
{
c = bmap.GetPixel(i, j);
int cR = c.R + brightness;
int cG = c.G + brightness;
int cB = c.B + brightness;
if (cR < 0) cR = 1;
if (cR > 255) cR = 255;
if (cG < 0) cG = 1;
if (cG > 255) cG = 255;
if (cB < 0) cB = 1;
if (cB > 255) cB = 255;
bmap.SetPixel(i, j, Color.FromArgb((byte)cR, (byte)cG, (byte)cB));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
4.Contrast(对比度)
对比比率是屏幕上同一点最亮时(白色)与最暗时(黑色)的亮度的比值,高的对比度意味着相对较高的亮度和呈现颜色的艳丽程度。
品质优异的LCD显示器面板和优秀的背光源亮度,两者合理配合就能获得色彩饱满明亮清晰的画面。
![]() | ![]() |
/// 设置对比度
/// </summary>
/// <param name="contrast">对比度,-100到+100之间的数值</param>
public void SetContrast(double contrast)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
if (contrast < -100) contrast = -100;
if (contrast > 100) contrast = 100;
contrast = (100.0 + contrast) / 100.0;
contrast *= contrast;
Color c;
for (int i = 0; i < bmap.Width; i++)
{
for (int j = 0; j < bmap.Height; j++)
{
c = bmap.GetPixel(i, j);
double pR = c.R / 255.0;
pR -= 0.5;
pR *= contrast;
pR += 0.5;
pR *= 255;
if (pR < 0) pR = 0;
if (pR > 255) pR = 255;
double pG = c.G / 255.0;
pG -= 0.5;
pG *= contrast;
pG += 0.5;
pG *= 255;
if (pG < 0) pG = 0;
if (pG > 255) pG = 255;
double pB = c.B / 255.0;
pB -= 0.5;
pB *= contrast;
pB += 0.5;
pB *= 255;
if (pB < 0) pB = 0;
if (pB > 255) pB = 255;
bmap.SetPixel(i, j, Color.FromArgb((byte)pR, (byte)pG, (byte)pB));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
5. Grayscale(灰度)
灰度使用黑色调表示物体。 每个灰度对象都具有从 0%(白色)到 100%(黑色)的亮度值。 使用黑白或灰度扫描仪生成的图像通常以灰度显示。
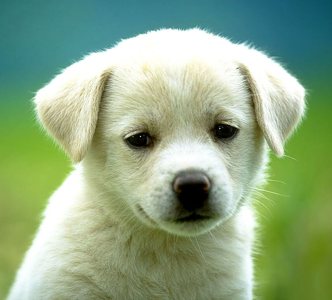
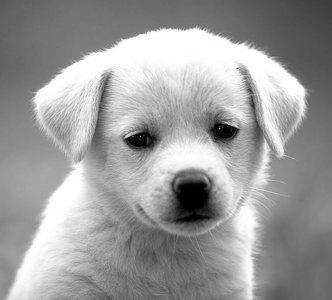
/// 设置灰度
/// </summary>
public void SetGrayscale()
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Color c;
for (int i = 0; i < bmap.Width; i++)
{
for (int j = 0; j < bmap.Height; j++)
{
c = bmap.GetPixel(i, j);
byte gray = (byte)(.299 * c.R + .587 * c.G + .114 * c.B);
bmap.SetPixel(i, j, Color.FromArgb(gray, gray, gray));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
6. Invert(底片效果)
用255减去每个色彩元素的值。例如,当前像素红色为00,则设置其值为FF(FF=255-0)。
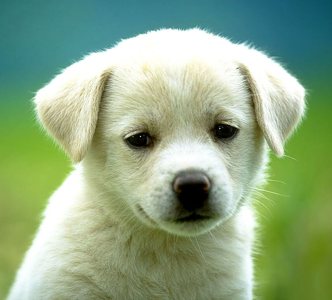

/// 底片
/// </summary>
public void SetInvert()
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Color c;
for (int i = 0; i < bmap.Width; i++)
{
for (int j = 0; j < bmap.Height; j++)
{
c = bmap.GetPixel(i, j);
bmap.SetPixel(i, j, Color.FromArgb(255 - c.R, 255 - c.G, 255 - c.B));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
7. Resize(缩放)
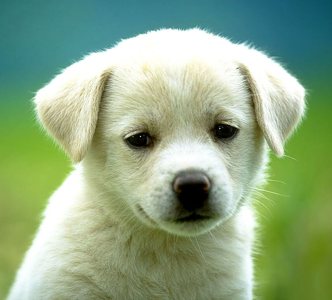
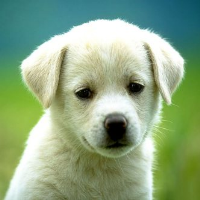
/// 缩放
/// </summary>
/// <param name="newWidth">宽</param>
/// <param name="newHeight">高</param>
public void Resize(int newWidth, int newHeight)
{
if (newWidth != 0 && newHeight != 0)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = new Bitmap(newWidth, newHeight, temp.PixelFormat);
double nWidthFactor = (double)temp.Width / (double)newWidth;
double nHeightFactor = (double)temp.Height / (double)newHeight;
double fx, fy, nx, ny;
int cx, cy, fr_x, fr_y;
Color color1 = new Color();
Color color2 = new Color();
Color color3 = new Color();
Color color4 = new Color();
byte nRed, nGreen, nBlue;
byte bp1, bp2;
for (int x = 0; x < bmap.Width; ++x)
{
for (int y = 0; y < bmap.Height; ++y)
{
fr_x = (int)Math.Floor(x * nWidthFactor);
fr_y = (int)Math.Floor(y * nHeightFactor);
cx = fr_x + 1;
if (cx >= temp.Width) cx = fr_x;
cy = fr_y + 1;
if (cy >= temp.Height) cy = fr_y;
fx = x * nWidthFactor - fr_x;
fy = y * nHeightFactor - fr_y;
nx = 1.0 - fx;
ny = 1.0 - fy;
color1 = temp.GetPixel(fr_x, fr_y);
color2 = temp.GetPixel(cx, fr_y);
color3 = temp.GetPixel(fr_x, cy);
color4 = temp.GetPixel(cx, cy);
// Blue
bp1 = (byte)(nx * color1.B + fx * color2.B);
bp2 = (byte)(nx * color3.B + fx * color4.B);
nBlue = (byte)(ny * (double)(bp1) + fy * (double)(bp2));
// Green
bp1 = (byte)(nx * color1.G + fx * color2.G);
bp2 = (byte)(nx * color3.G + fx * color4.G);
nGreen = (byte)(ny * (double)(bp1) + fy * (double)(bp2));
// Red
bp1 = (byte)(nx * color1.R + fx * color2.R);
bp2 = (byte)(nx * color3.R + fx * color4.R);
nRed = (byte)(ny * (double)(bp1) + fy * (double)(bp2));
bmap.SetPixel(x, y, System.Drawing.Color.FromArgb(255, nRed, nGreen, nBlue));
}
}
_currentBitmap = (Bitmap)bmap.Clone();
}
}
8. Rotating and Flipping(镜像)
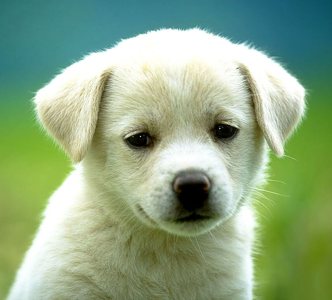

/// 翻转
/// </summary>
/// <param name="rotateFlipType">图像的旋转方向和用于翻转图像的轴。</param>
public void RotateFlip(RotateFlipType rotateFlipType)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
bmap.RotateFlip(rotateFlipType);
_currentBitmap = (Bitmap)bmap.Clone();
}
9. Crop(裁剪)
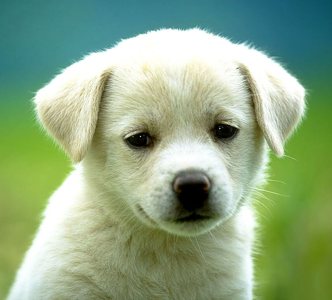
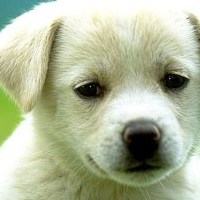
/// 裁剪
/// </summary>
/// <param name="xPosition">X起始点</param>
/// <param name="yPosition">Y起始点</param>
/// <param name="width">宽</param>
/// <param name="height">高</param>
public void DrawOutCropArea(int xPosition, int yPosition, int width, int height)
{
_bitmapPrevCropArea = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)_bitmapPrevCropArea.Clone();
Graphics gr = Graphics.FromImage(bmap);
Brush cBrush = new Pen(Color.FromArgb(150, Color.White)).Brush;
Rectangle rect1 = new Rectangle(0, 0, _currentBitmap.Width, yPosition);
Rectangle rect2 = new Rectangle(0, yPosition, xPosition, height);
Rectangle rect3 = new Rectangle(0, (yPosition + height), _currentBitmap.Width, _currentBitmap.Height);
Rectangle rect4 = new Rectangle((xPosition + width), yPosition, (_currentBitmap.Width - xPosition - width), height);
gr.FillRectangle(cBrush, rect1);
gr.FillRectangle(cBrush, rect2);
gr.FillRectangle(cBrush, rect3);
gr.FillRectangle(cBrush, rect4);
_currentBitmap = (Bitmap)bmap.Clone();
}
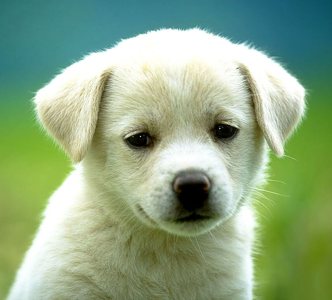
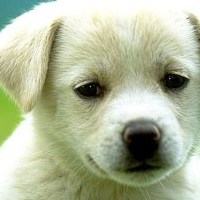
/// 裁剪
/// </summary>
/// <param name="xPosition">X点位置</param>
/// <param name="yPosition">Y点位置</param>
/// <param name="width">宽度</param>
/// <param name="height">高度</param>
public void Crop(int xPosition, int yPosition, int width, int height)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
if (xPosition + width > _currentBitmap.Width)
width = _currentBitmap.Width - xPosition;
if (yPosition + height > _currentBitmap.Height)
height = _currentBitmap.Height - yPosition;
Rectangle rect = new Rectangle(xPosition, yPosition, width, height);
_currentBitmap = (Bitmap)bmap.Clone(rect, bmap.PixelFormat);
}
10. Inserting Text, Any Other Images and Shapes(插入文字或其它图像或形状)
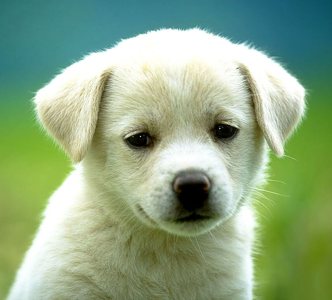
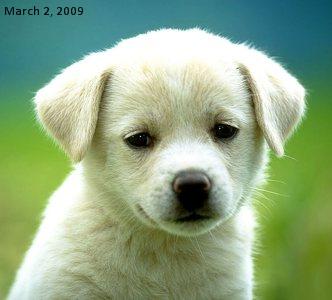
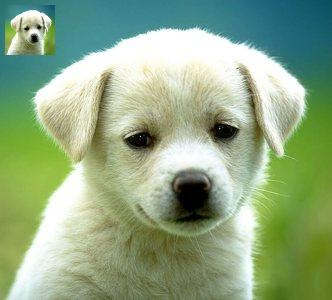
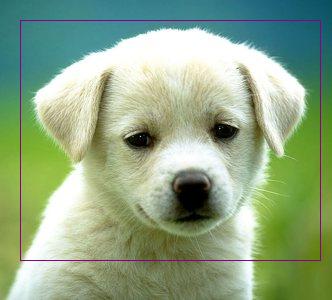
/// 插入文字
/// </summary>
/// <param name="text">要插入的文字</param>
/// <param name="xPosition">X位置</param>
/// <param name="yPosition">Y位置</param>
/// <param name="fontName">字体</param>
/// <param name="fontSize">大小</param>
/// <param name="fontStyle">类型</param>
/// <param name="colorName1">颜色</param>
/// <param name="colorName2">颜色</param>
public void InsertText(string text, int xPosition, int yPosition, string fontName, float fontSize, string fontStyle, string colorName1, string colorName2)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Graphics gr = Graphics.FromImage(bmap);
if (string.IsNullOrEmpty(fontName))
fontName = "Times New Roman";
if (fontSize.Equals(null))
fontSize = 10.0F;
Font font = new Font(fontName, fontSize);
if (!string.IsNullOrEmpty(fontStyle))
{
FontStyle fStyle = FontStyle.Regular;
switch (fontStyle.ToLower())
{
case "bold":
fStyle = FontStyle.Bold;
break;
case "italic":
fStyle = FontStyle.Italic;
break;
case "underline":
fStyle = FontStyle.Underline;
break;
case "strikeout":
fStyle = FontStyle.Strikeout;
break;
}
font = new Font(fontName, fontSize, fStyle);
}
if (string.IsNullOrEmpty(colorName1))
colorName1 = "Black";
if (string.IsNullOrEmpty(colorName2))
colorName2 = colorName1;
Color color1 = Color.FromName(colorName1);
Color color2 = Color.FromName(colorName2);
int gW = (int)(text.Length * fontSize);
gW = gW == 0 ? 10 : gW;
LinearGradientBrush LGBrush = new LinearGradientBrush(new Rectangle(0, 0, gW, (int)fontSize), color1, color2, LinearGradientMode.Vertical);
gr.DrawString(text, font, LGBrush, xPosition, yPosition);
_currentBitmap = (Bitmap)bmap.Clone();
}
插入图像
/// 插入图像
/// </summary>
/// <param name="imagePath">要插入的图像路径</param>
/// <param name="xPosition">X位置</param>
/// <param name="yPosition">Y位置</param>
public void InsertImage(string imagePath, int xPosition, int yPosition)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Graphics gr = Graphics.FromImage(bmap);
if (!string.IsNullOrEmpty(imagePath))
{
Bitmap i_bitmap = (Bitmap)Bitmap.FromFile(imagePath);
Rectangle rect = new Rectangle(xPosition, yPosition, i_bitmap.Width, i_bitmap.Height);
gr.DrawImage(Bitmap.FromFile(imagePath), rect);
}
_currentBitmap = (Bitmap)bmap.Clone();
}
插入图形
/// 插入形状
/// </summary>
/// <param name="shapeType"></param>
/// <param name="xPosition"></param>
/// <param name="yPosition"></param>
/// <param name="width"></param>
/// <param name="height"></param>
/// <param name="colorName"></param>
public void InsertShape(string shapeType, int xPosition, int yPosition, int width, int height, string colorName)
{
Bitmap temp = (Bitmap)_currentBitmap;
Bitmap bmap = (Bitmap)temp.Clone();
Graphics gr = Graphics.FromImage(bmap);
if (string.IsNullOrEmpty(colorName))
colorName = "Black";
Pen pen = new Pen(Color.FromName(colorName));
switch (shapeType.ToLower())
{
case "filledellipse":
gr.FillEllipse(pen.Brush, xPosition, yPosition, width, height);
break;
case "filledrectangle":
gr.FillRectangle(pen.Brush, xPosition, yPosition, width, height);
break;
case "ellipse":
gr.DrawEllipse(pen, xPosition, yPosition, width, height);
break;
case "rectangle":
default:
gr.DrawRectangle(pen, xPosition, yPosition, width, height);
break;
}
_currentBitmap = (Bitmap)bmap.Clone();
}
翻译转自CSDN: http://topic.csdn.net/u/20090420/00/4042e404-e802-45f7-8b25-c7fbc5a81c76.html本文来源: